📖 개요
📌 오늘 할 일
◻ 프로그램 정보 창 CSS
◻ 내 PC Hostname 가져오기
◻ 내 PC IP 가져오기
완성된 화면 보기 👉 Electron
📁 폴더 구조
💻 프로그램 정보 CSS (display:grid)
.grid{
display: grid;
grid-template-columns: 1fr 1fr;
grid-template-rows: 200px auto;
align-items: center;
text-align: center;
}
grid-template-* 행(row), 열(columns)가 있다.
우선 columns를 1:1로 나누었다. (1fr 1fr)
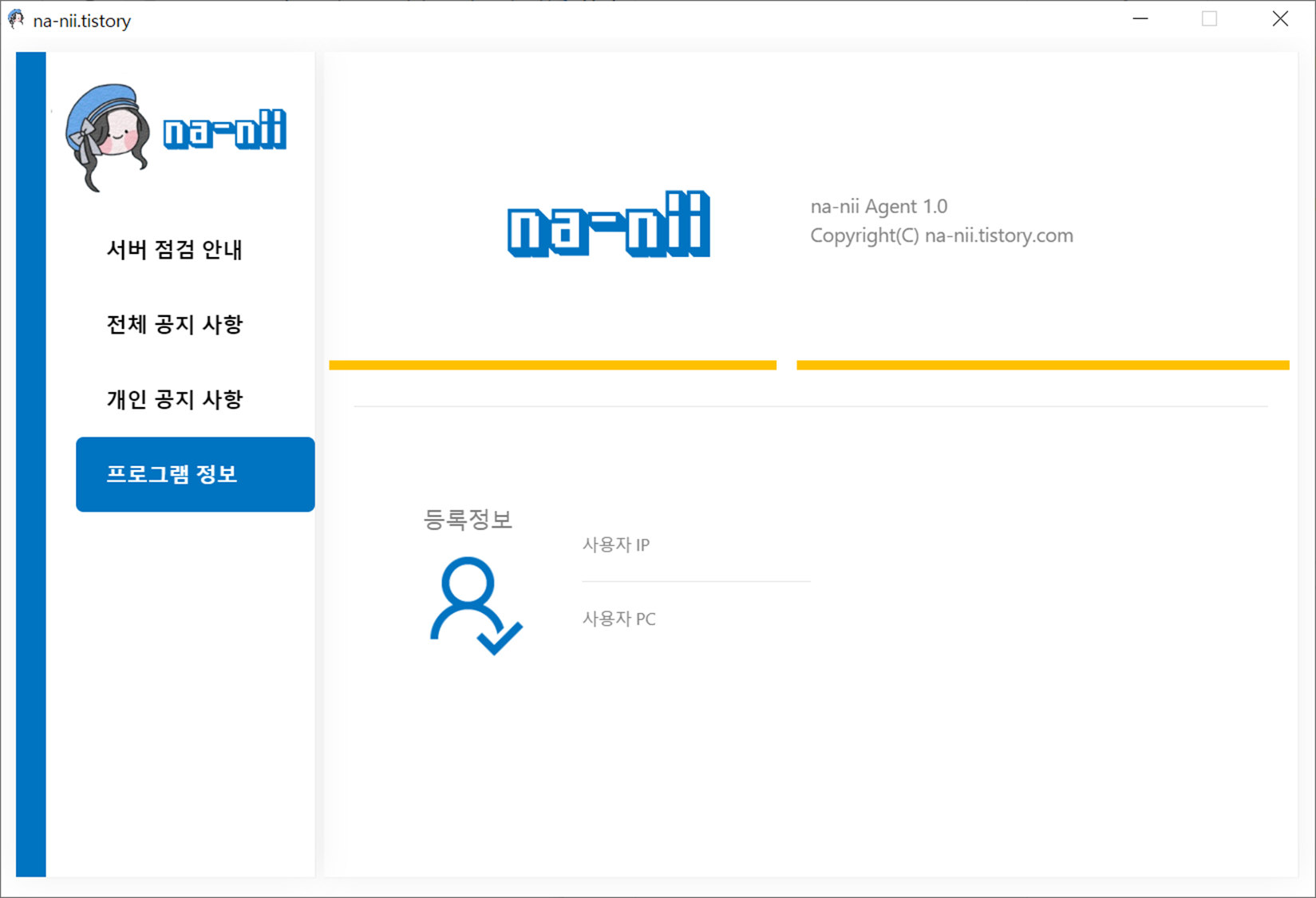
row를 200px 하고 나머지(*)를 할당한 것을 알 수 있다.
전체 코드
//info.js
import React from 'react';
import Logo from '../image/na-nii.png';
import user from '../image/user4.png'
const text = {0: 'na-nii Agent 1.0\n Copyright(C) na-nii.tistory.com'}
const Info = () => {
return (
<div className='list'>
<div className='grid'>
<img src={ Logo } className="Logo_info" alt= "Logo" width='200'/>
<p className='text'> {text[0]} <br /></p>
</div>
<hr width="100%" />
<div className='grid' >
<div className='grid'>
<div>
<div className='text1'>등록정보</div>
<img src={user} alt= "Logo" width='80'/>
</div>
<div>
<div className='text_title'> 사용자 IP </div>
<hr />
<div className='text_title'> 사용자 PC </div>
</div>
</div>
</div>
</div>
);
};
export default Info;
//detail.css
/***************************
Info.jsx
***************************/
.grid{
display: grid;
grid-template-columns: 1fr 1fr;
grid-template-rows: 200px auto;
align-items: center;
text-align: center;
}
.Logo_info /* 로고 위치 */
{
margin-left:70px;
}
.text_title{ /* 사용자 IP, 사용자 PC */
color: gray;
text-align: left;
font-size: 11px;
white-space: pre-line;
}
.text{ /* pcName */
color: gray;
text-align: left;
font-size: 13px;
white-space: pre-line;
}
.text1{ /*등록정보*/
color: gray;
text-align: center;
font-size: 15px;
white-space: pre-line;
}
💻 내 PC hostname 가져오기
node.js의 내장모듈 중 'os'를 사용해서 hostname을 가져올 것이다.
const os = window.require('os');
const pcName = os.userInfo().username;
<div className='text'> {pcName} </div>
window.require 함수를 쓰게 되면 웹페이지에서 window.require 에러가 뜬다.
이건 무시하고 electron 앱에서 뜨는 지 확인하면 된다. 만약 electron 앱에서도 동일한 에러가 뜨면 main.js 파일에 contextIsoloation: false를 추가하면 된다. 자세한 건 이전 포스팅을 참고하자!
💻 내 PC hostname 가져오기
ip 모듈을 설치한다.
npm i ip
ip 모듈을 사용해서 IP를 가져올 수 있다.
const ip = window.require('ip')
const ipaddr = ip.address();
<div className='text'> {ipaddr} </div>
만약 모듈을 안 쓰고 싶으면 소스코드를 사용하는 방법도 있다.
//IP.js
import React from 'react';
const IP = () => {
var RTCPeerConnection = /*window.RTCPeerConnection ||*/ window.webkitRTCPeerConnection || window.mozRTCPeerConnection;
if (RTCPeerConnection)(function() {
var rtc = new RTCPeerConnection({
iceServers: []
});
if (1 || window.mozRTCPeerConnection) {
rtc.createDataChannel('', {
reliable: false
});
};
rtc.onicecandidate = function(evt) {
if (evt.candidate) grepSDP("a=" + evt.candidate.candidate);
};
rtc.createOffer(function(offerDesc) {
grepSDP(offerDesc.sdp);
rtc.setLocalDescription(offerDesc);
}, function(e) {
console.warn("offer failed", e);
});
var addrs = Object.create(null);
addrs["0.0.0.0"] = false;
function updateDisplay(newAddr) {
if (newAddr in addrs) return;
else addrs[newAddr] = true;
var displayAddrs = Object.keys(addrs).filter(function(k) {
return addrs[k];
});
document.getElementById('list').textContent = displayAddrs.join(" or ") || "n/a";
}
function grepSDP(sdp) {
var hosts = [];
sdp.split('\r\n').forEach(function(line) {
if (~line.indexOf("a=candidate")) {
var parts = line.split(' '),
addr = parts[4],
type = parts[7];
if (type === 'host') updateDisplay(addr);
} else if (~line.indexOf("c=")) {
var parts = line.split(' '),
addr = parts[2];
updateDisplay(addr);
}
});
}
})();
else {
document.getElementById('list').innerHTML = "<code>ifconfig| grep inet | grep -v inet4 | cut -d\" \" -f2 | tail -n1</code>";
document.getElementById('list').nextSibling.textContent = "In Chrome and Firefox your IP should display automatically, by the power of WebRTCskull.";
}
return (
<div className='text' id="list"></div>
);
};
export default IP;
//info.js
import IP from '../components/IP'
<IP/>
인터넷이 되는 환경이라면 CDN을 통해서 가져오는 다양한 방법이 있다. (지역, 위치까지도 가져올 수 있다.) 하지만 사내 내부망에서 동작하는 데스크톱 어플리케이션이라 인터넷 없이 IP를 가져와야해서 번거롭지만 모듈이나 소스를 사용해야 했다.
💻 전체 코드
//info.js
import React from 'react';
// import IP from '../components/IP'
import Logo from '../image/na-nii.png';
import user from '../image/user4.png'
const text = {0: 'na-nii Agent 1.0\n Copyright(C) na-nii.tistory.com'}
const Info = () => {
const os = window.require('os')
const pcName = os.userInfo().username;
const ip = window.require('ip')
const ipaddr = ip.address();
return (
<div className='list'>
<div className='grid'>
<img src={ Logo } className="Logo_info" alt= "Logo" width='200'/>
<p className='text'> {text[0]} <br /></p>
</div>
<hr width="100%" />
<div className='grid' >
<div className='grid'>
<div>
<div className='text1'>등록정보</div>
<img src={user} alt= "Logo" width='80'/>
</div>
<div>
<div className='text_title'> 사용자 IP </div>
{/* <IP /> */}
<div className='text'> {ipaddr} </div>
<hr />
<div className='text_title'> 사용자 PC </div>
<div className='text'> {pcName} </div>
</div>
</div>
</div>
</div>
);
};
export default Info;
📌 참고 사이트
Getting Client IP Address Or Local IP Address In JavaScript
Have a look at this blog to learn how can we get the local IP address of a user in JavaScript. Getting Client IP Address Or Local IP Address In JavaScript. So, it all started with a requirement for my website when I needed to get the client's IP address fo
morioh.com
'💻 프로젝트 > 02. 사내 공지 데스크톱 앱' 카테고리의 다른 글
『Client 04 』 [React] 게시판 만들기 -BoardList, props 전달, custom axios (0) | 2023.09.19 |
---|---|
『Server 04 』[express] [mysql2] Node.js 서버 개설, DB 연동(GET) (0) | 2023.09.17 |
『Client 02 』 [React] [Electron] React 사이드 메뉴, Electron Icon 변경하기 (0) | 2023.09.16 |
『Client 01 』 [React] [Electron] Electron에 React 창 띄우기 (0) | 2023.09.16 |
『Server 03 』 [React] 프로젝트 생성 + 불필요한 파일 정리 (0) | 2023.09.13 |